Looking for an identity bootstrapping tool for ASP.NET Core? Check out Getting Started with IdentityManager2 for ASP.NET Core Identity and IdentityServer4 support.
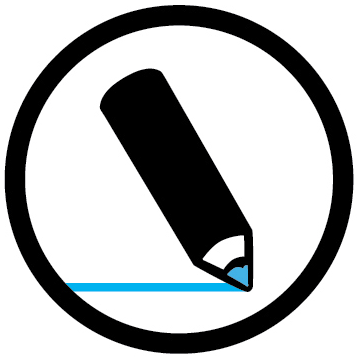
Identity Manager (formerly Thinktecture Identity Manager) is the spiritual successor to the ASP.NET Web Site Administration Tool that used to be available with Visual Studio, providing a simple UI for performing CRUD operations to manage your user store.
IdentityManager is a tool for developers and/or administrators to manage the identity information for users of their applications. This includes creating users, editing user information (passwords, email, claims, etc.) and deleting users. It provides a modern replacement for the ASP.NET WebSite Administration tool that used to be built into Visual Studio. - https://github.com/IdentityManager/IdentityManager
Created by Brock Allen of Identity Server and Identity Model fame, Identity Manager uses a RESTful API that abstracts the underlying Identity database, exposing metadata and functionality that powers a browser-based UI or used programmatically within your software.
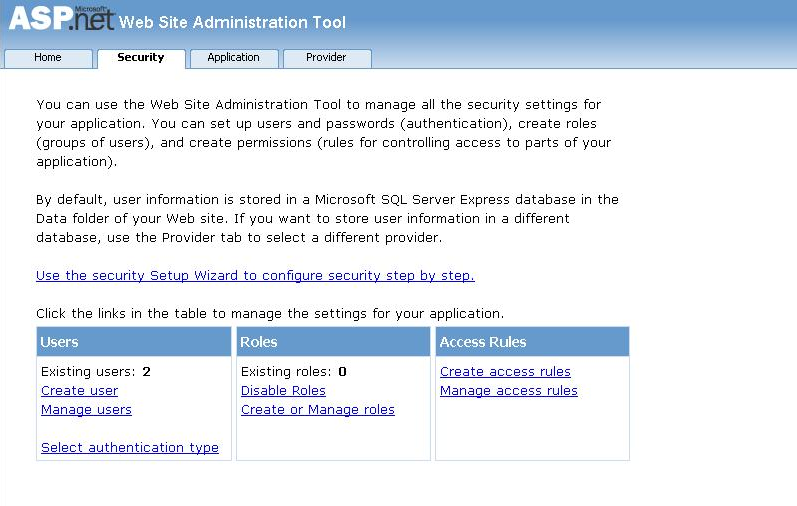
ASP.NET Web Site Administration Tool - Wikipedia

Identity Manager
Identity Manager currently has out-of-the-box support for ASP.NET Identity v2 and MembershipReboot (another project from Brock Allen, this time modernizing concepts from the old ASP.NET Membership system and available as an alternative to ASP.NET Identity).
Much like Identity Server, Identity Manager is a OWIN/Katana middleware and can be configured to run in any OWIN host and in turn can use any Katana based authentication middleware for authentication.
Identity Manager using ASP.NET Identity
Installation
To demonstrate Identity Manager we’ll create an example implementation using ASP.NET Identity v2 as our user store.
We’ll start off with an empty ASP.NET application, no MVC or Authentication templates will be needed here. To start off you’ll need the following packages:
Install-Package IdentityManager -Pre
Install-Package IdentityManager.AspNetIdentity -Pre
Do note that these packages pull down Owin
and Microsoft.AspNet.Identity.Core
as dependencies.
For our example we will use the EF implementation of ASP.NET Identity and run the site on IIS so we’ll need to also install:
Install-Package Microsoft.AspNet.Identity.EntityFramework
Install-Package Microsoft.Owin.Host.SystemWeb
IdentityManagerService
An implementation of the IIdentityManagerService
abstracts out the underlying Identity management library for use with Identity Manager.
In our case this will be ASP.NET Identity.
To keep things simple, we’ll new up some Store and Manager classes using the default IdentityUser
and IdentityRole
types and register these with Identity Manager, using a simple factory method to resolve them per request.
private IIdentityManagerService Create() {
var context =
new IdentityDbContext(
@"Data Source=.\SQLEXPRESS;Initial Catalog=AspIdentity;Integrated Security=true");
var userStore = new UserStore<IdentityUser>(context);
var userManager = new UserManager<IdentityUser>(userStore);
var roleStore = new RoleStore<IdentityRole>(context);
var roleManager = new RoleManager<IdentityRole>(roleStore);
var managerService =
new AspNetIdentityManagerService<IdentityUser, string, IdentityRole, string>
(userManager, roleManager);
return managerService;
}
Once we have done this we simply need to register the Identity Server katana component in our OWIN startup class:
public void Configuration(IAppBuilder app) {
var factory = new IdentityManagerServiceFactory();
factory.IdentityManagerService =
new Registration<IIdentityManagerService>(Create());
app.UseIdentityManager(new IdentityManagerOptions { Factory = factory });
}
Here we are initialising the Identity Manager DI container with our IdentityManagerService
and then adding Identity Manager to our OWIN pipeline.
Again as with Identity Server, we’ll need to enable RAMMFAR in your web.config when hosting in IIS, in order for Identity Manager’s embedded resources to be loaded correctly (otherwise you’ll most likely be met with a blank screen).
<system.webServer>
<modules runAllManagedModulesForAllRequests="true" />
</system.webServer>
In order to run Identity Manager, you will also need to assign it a HTTPS URL. Identity Manager will not run over HTTP out of the box.
And that’s all there is to it!

Identity Manager Security using Identity Server
Securing Identity Manager is pretty simple when using Identity Server as it is treated in the same way as any other OpenID Connect application. We could use other authentication platforms or protocols but we all love Identity Server and it is, after all, an OpenID Connect Provider.
To initially configure Identity Server check out Part 1 of my Identity Server Implementation Guide. The following example will assume you have a working Identity Server implementation and will detail the necessary Clients and Scopes to secure Identity Manager.
Before we start make sure you add a user to the IdentityManagerAdministrator
role so that you are authorized access to Identity Manager.
Scope
First of all we’ll add a custom scope for accessing Identity Manager and at the same time ensure it always receives the scopes it needs. Add the following Scope
entity to your scope store:
new Scope {
Name = "identity_manager",
DisplayName = "Identity Manager",
Description = "Authorization for Identity Manager",
Type = ScopeType.Identity,
Claims = new List<ScopeClaim> {
new ScopeClaim(Constants.ClaimTypes.Name),
new ScopeClaim(Constants.ClaimTypes.Role)
}
}
Client
We’ll now need to add the following Client
entity to our client store:
new Client {
ClientId = "identity_manager",
ClientName = "Identity Manager",
Enabled = true,
RedirectUris = new List<string> {"https://localhost:44307/"},
Flow = Flows.Implicit,
RequireConsent = false,
AllowedScopes = new List<string> {
Constants.StandardScopes.OpenId,
"identity_manager"
}
}
Identity Manager
To add OpenID Connect authentication to our Identity Manager installation, we’ll need the following packages:
Install-Package Microsoft.Owin.Security.Cookies
Install-Package Microsoft.Owin.Security.OpenIdConnect
We’ll then need to add the following to the beginning of our OWIN Startup class:
JwtSecurityTokenHandler.InboundClaimTypeMap = new Dictionary<string, string>();
app.UseCookieAuthentication
(new CookieAuthenticationOptions {AuthenticationType = "cookies"});
app.UseOpenIdConnectAuthentication(new OpenIdConnectAuthenticationOptions {
AuthenticationType = "oidc",
Authority = "https://localhost:44300/Core/",
ClientId = "identity_manager",
RedirectUri = "https://localhost:44307/",
ResponseType = "id_token",
UseTokenLifetime = true,
Scope = "openid identity_manager",
SignInAsAuthenticationType = "cookies"
});
Finally, we also need to set the SecurityConfiguration
property of our IdentityManagerOptions
:
SecurityConfiguration = new HostSecurityConfiguration {
HostAuthenticationType = "cookies",
AdditionalSignOutType = "oidc"
}
Once this is done we will now be required to authenticate whenever we use Identity Manager and must log in using an Identity with the IdentityManagerAdministrator role claim.
Note that Identity Manager is currently still in beta. The development team is currently primarily focused on Identity Server but the project is good for use by developers and testers. I’ll keep this article up to date with any breaking changes.
Resources
If you are have any issues with setting up Identity Manager or just prefer a video tutorial, check out Brock Allen's video IdentityManager with ASP.NET Identity on Vimeo:
There is also a similar tutorial for securing Identity Manager using Identity Server.
Source Code
I've uploaded a simple example of Identity Manager running using ASP.NET Identity on GitHub. I've kept this example as simple as possible so that it's only a few lines of code. If anyone has any issues with securing Identity Manager using Identity Server, let me know in the comments below and I'll look at uploading a working copy of both.